In JavaScript, objects are a fundamental data type used to store collections of key-value pairs. Often, developers find themselves in situations where they need to combine the properties of two or more objects into a single object.
Understanding how to js merge objects effectively is crucial for maintaining clean and efficient code. This article will explore several common scenarios and methods for merging object properties in JavaScript.
Method 1 ─ Using Object.assign()
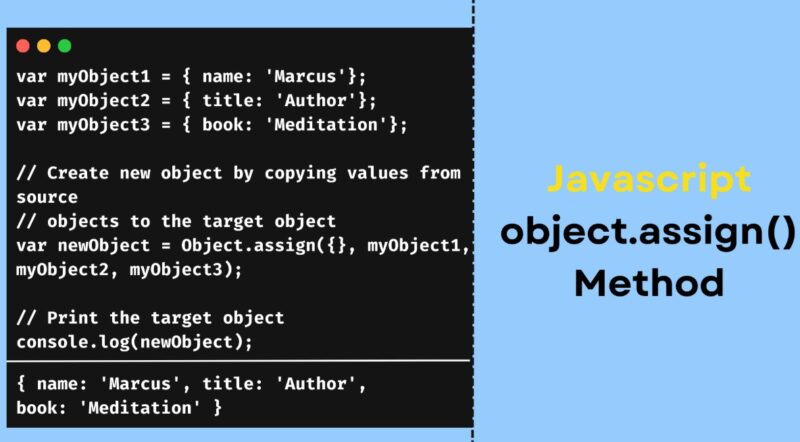
Object.assign() is a built-in JavaScript method that allows you to copy the values of all enumerable properties from one or more source objects to a target object. The basic syntax is as follows:
Object.assign(target, source1, source2, …)
Here’s an example of how to use Object.assign() to merge two objects:
const obj1 = { a: 1, b: 2 };
const obj2 = { c: 3, d: 4 };
const mergedObj = Object.assign({}, obj1, obj2);
console.log(mergedObj); // Output: { a: 1, b: 2, c: 3, d: 4 }
Pros:
- Simple and straightforward syntax
- Supported in most modern browsers
Cons:
- Does not perform a deep merge (nested objects are not merged recursively)
- If source objects have the same property keys, the last source object’s value will overwrite the previous ones
Method 2 ─ Using the Spread Operator (…)
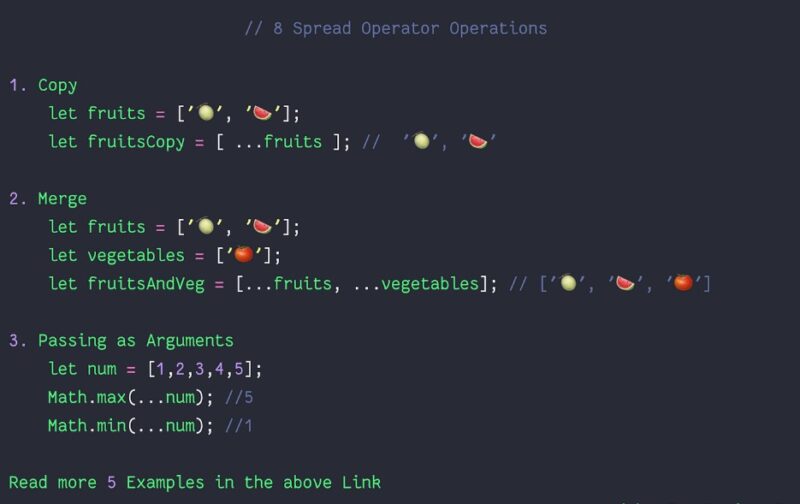
The spread operator (…), introduced in ECMAScript 6 (ES6), provides a concise way to merge objects. It allows you to spread the properties of an object into another object. Here’s an example:
const obj1 = { a: 1, b: 2 };
const obj2 = { c: 3, d: 4 };
const mergedObj = { …obj1, …obj2 };
console.log(mergedObj); // Output: { a: 1, b: 2, c: 3, d: 4 }
Pros:
- Concise and readable syntax
- Supports a shallow merge of objects
Cons:
- Not supported in older browsers (requires ES6 support)
- Like Object.assign(), if source objects have the same property keys, the last source object’s value will overwrite the previous ones
Method 3 ─ Manual Property Assignment with a Loop
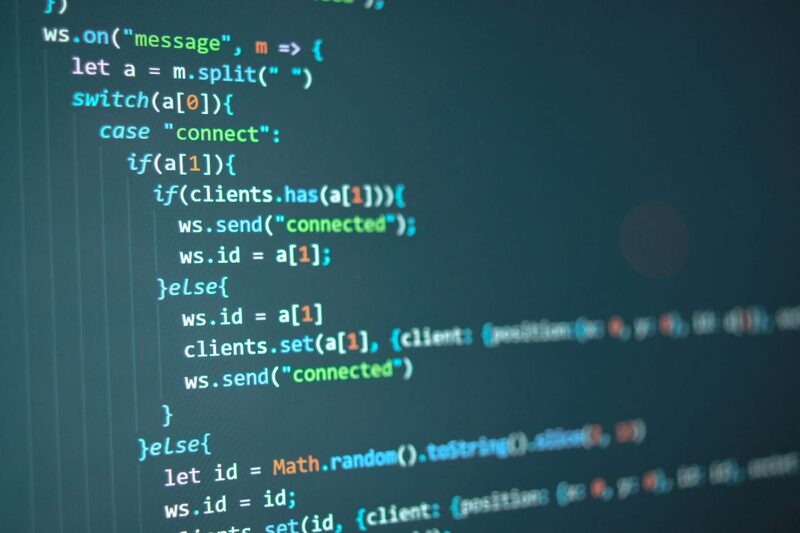
In some cases, you may need more control over the merging process or want to include conditional logic. You can achieve this by manually iterating over the object properties and assigning them to the target object. Here’s an example:
function mergeObjects(obj1, obj2) {
const mergedObj = {};
for (let key in obj1) {
mergedObj[key] = obj1[key];
}
for (let key in obj2) {
mergedObj[key] = obj2[key];
}
return mergedObj;
}
const obj1 = { a: 1, b: 2 };
const obj2 = { c: 3, d: 4 };
const mergedObj = mergeObjects(obj1, obj2);
console.log(mergedObj); // Output: { a: 1, b: 2, c: 3, d: 4 }
Pros:
- Provides more control over the merging process
- Allows for conditional logic or custom merging behavior
Cons:
- Requires more code compared to using built-in methods or operators
- Manual property assignment can be error-prone if not handled carefully
Recursive Merging of Nested Objects
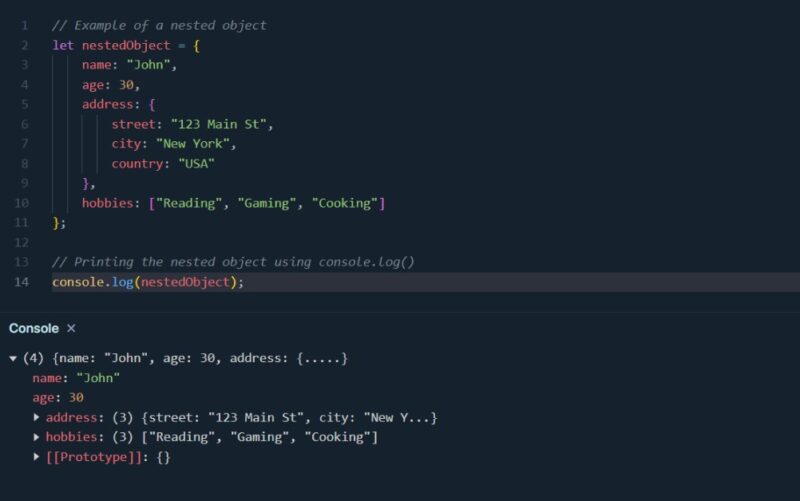
When dealing with objects that contain nested objects or complex structures, you may need to perform a deep merge. A deep merge recursively merges the properties of nested objects. Here’s an example of a recursive function that performs a deep merge:
function deepMerge(target, source) {
for (let key in source) {
if (typeof source[key] === ‘object’ && source[key] !== null) {
if (!(key in target)) {
target[key] = {};
}
deepMerge(target[key], source[key]);
} else {
target[key] = source[key];
}
}
return target;
}
const obj1 = { a: 1, b: { c: 2 } };
const obj2 = { b: { d: 3 }, e: 4 };
const mergedObj = deepMerge({}, obj1);
deepMerge(mergedObj, obj2);
console.log(mergedObj); // Output: { a: 1, b: { c: 2, d: 3 }, e: 4 }
When performing a deep merge, it’s important to consider the following:
- Ensure that the target object and its nested objects are properly initialized to avoid errors.
- Be cautious when merging objects with circular references, as it can lead to infinite recursion.
Comparison of Object Merging Methods
Method | Browser Support | Performance | Readability | Deep Merge | Latenode Compatibility |
Object.assign() | Good | Fast | Good | No | High |
Spread Operator (…) | ES6+ | Fast | Excellent | No | High |
Manual Loop | All | Slow | Fair | Yes | Medium |
Recursive Function | All | Slow | Fair | Yes | Medium |
As seen in the comparison table above, Object.assign() and the spread operator (…) are highly compatible with the Latenode automation platform. They offer good performance and readability, making them the preferred choices for object merging in Latenode projects. However, they do not support deep merging out of the box.
On the other hand, manual loops and recursive functions provide more flexibility and support deep merging but may have slower performance and reduced readability. They have medium compatibility with Latenode, requiring additional implementation efforts.
Practical Examples of Object Merging
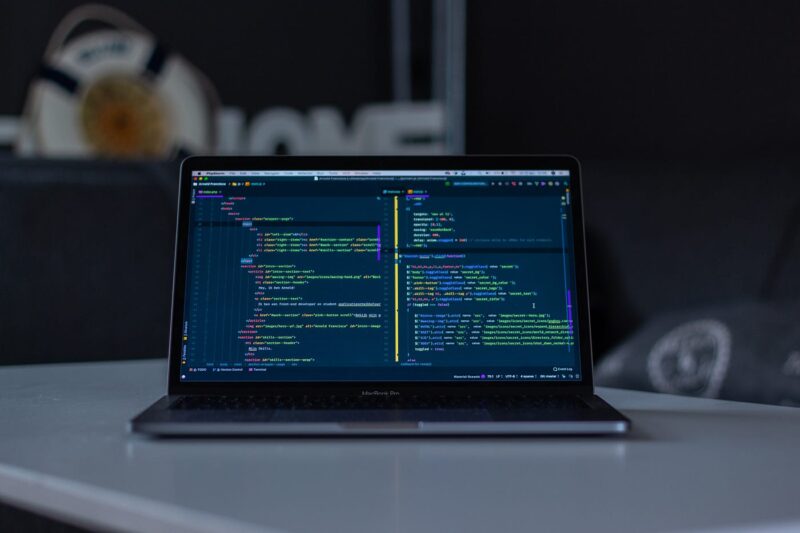
Merging Configuration Settings
Suppose you have a default configuration object and want to merge it with user-provided settings. Object merging allows you to create a new configuration object that combines the default values with the user overrides.
const defaultConfig = {
apiUrl: ‘https://api.example.com’,
timeout: 5000,
retries: 3
};
const userConfig = {
timeout: 10000,
maxResults: 100
};
const finalConfig = { …defaultConfig, …userConfig };
console.log(finalConfig);
1. // Output: { apiUrl: ‘https://api.example.com’, timeout: 10000, retries: 3, maxResults: 100 }
Combining Data from Multiple Sources
When working with data from multiple sources, object merging can be used to combine the data into a single object. This is particularly useful when dealing with APIs that return partial data or when aggregating information from different endpoints.
const userData = {
id: 1,
name: ‘John Doe’,
email: ‘john@example.com’
};
const userDetails = {
id: 1,
age: 30,
address: ‘123 Street, City’
};
const userProfile = { …userData, …userDetails };
console.log(userProfile);
2. // Output: { id: 1, name: ‘John Doe’, email: ‘john@example.com’, age: 30, address: ‘123 Street, City’ }
Performance and Optimization
When working with large objects or frequently merging objects, performance becomes a crucial consideration. Here are a few tips to optimize object merging:
1. Use <span style=”font-size: 11pt; font-family: ‘Roboto Mono’, monospace; color: rgb(24, 128, 56);